optimize Your Website Via Functions.php File
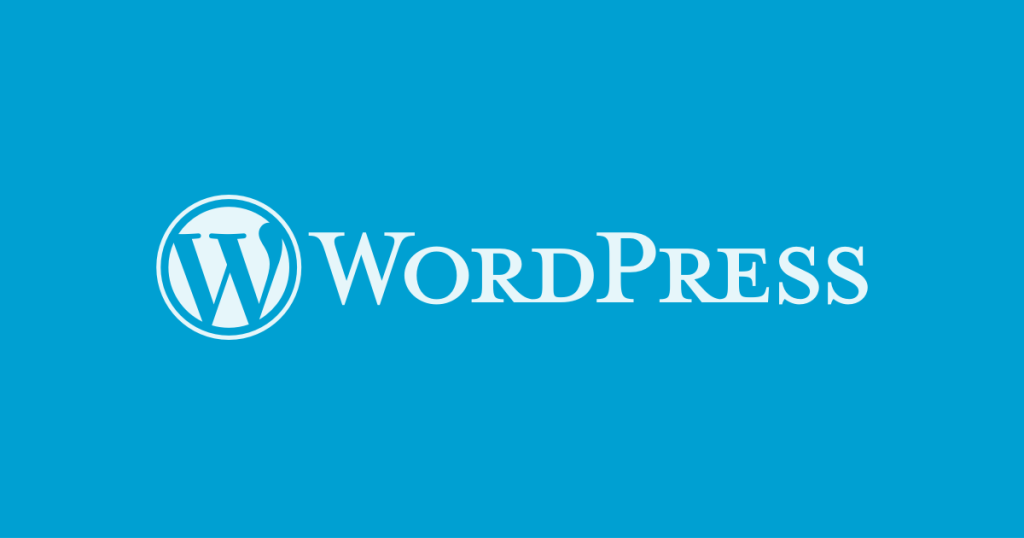
Optimizing a website through the functions.php
file in WordPress is a common practice to improve performance, functionality, and other aspects of your website. The functions.php
file is part of your theme and can be used to add custom code and functions to your WordPress site. Here are some optimization techniques you can implement using the functions.php
file:
Enqueue Scripts and Styles Properly: Use the wp_enqueue_script()
and wp_enqueue_style()
functions to load JavaScript and CSS files efficiently. This helps in proper script loading and reduces page load times.
function enqueue_custom_scripts() {
wp_enqueue_script('custom-script', get_template_directory_uri() . '/js/custom.js', array('jquery'), '1.0', true);
wp_enqueue_style('custom-style', get_stylesheet_uri());
}
add_action('wp_enqueue_scripts', 'enqueue_custom_scripts');
Enable Gzip Compression: Gzip compression reduces the size of files transferred between the server and the user’s browser. You can enable it using the following code:
function enable_gzip_compression() {
if (function_exists('ob_gzhandler')) {
ob_start('ob_gzhandler');
}
}
add_action('init', 'enable_gzip_compression');
Optimize Images: You can automatically compress and serve optimized images using plugins like “Smush” or by adding custom code to your functions.php
file. Be cautious when modifying images programmatically, as it might affect their quality.
Implement Caching: Caching helps store static content to reduce server load and improve page loading times. Use plugins like “W3 Total Cache” or “WP Super Cache” to handle caching, or implement it yourself using code.
Optimize Database Queries: Reduce the number of database queries and optimize the existing ones for better performance. Use the $wpdb
object for custom queries and make use of indexes and proper SQL queries.
Disable Emojis and Embeds: Remove unnecessary functionality like emojis and oEmbeds if you don’t need them. This can be done in the functions.php
file:
function disable_emojis() {
remove_action('wp_head', 'print_emoji_detection_script', 7);
remove_action('admin_print_scripts', 'print_emoji_detection_script');
remove_action('wp_print_styles', 'print_emoji_styles');
remove_action('admin_print_styles', 'print_emoji_styles');
remove_filter('the_content_feed', 'wp_staticize_emoji');
remove_filter('comment_text_rss', 'wp_staticize_emoji');
remove_filter('wp_mail', 'wp_staticize_emoji_for_email');
}
add_action('init', 'disable_emojis');
Limit Post Revisions: WordPress stores revisions of posts, which can increase the size of your database. Limit the number of revisions stored or disable them completely to reduce database bloat.
define('WP_POST_REVISIONS', 5); // Limit to 5 revisions
Remove Query Strings from Static Resources: Query strings in URLs can prevent proper caching. You can remove them using the following code:
function remove_query_strings() {
if (!is_admin()) {
add_filter('script_loader_src', 'remove_query_strings');
add_filter('style_loader_src', 'remove_query_strings');
}
}
add_action('init', 'remove_query_strings');
Remember to make a backup of your functions.php
file before making changes and test each modification on a staging site or local environment before implementing it on your live site. Incorrect code can lead to errors or even a site outage.
If you’re encountering a “Cannot redeclare” error, which occurs when a function is declared more than once in your code. The remove_query_strings()
function is being declared multiple times, which is causing the error.
This issue could arise if you have included the same code block in your functions.php
file more than once, or if you are using a child theme and the same function is declared in both the parent and child themes.
To resolve this issue, follow these steps:
Locate the Duplicate Declaration: In your
functions.php
files (both in the parent and child themes), locate where theremove_query_strings()
function is being declared. Make sure you have only one declaration of this function.Merge or Remove Duplicate Code: If you find multiple declarations of the
remove_query_strings()
function, choose one version to keep and remove the others. If you have a declaration in both the parent and child themes, it’s generally recommended to keep the child theme’s version.Check for Included Files: Sometimes, the same function might be included from external files, causing the duplication. Make sure you’re not including the same file more than once.
Use Conditional Checks: To avoid redeclaration issues, you can use conditional checks to ensure that a function is declared only once. For example:
if (!function_exists('remove_query_strings')) {
function remove_query_strings() {
// Function code here
}
}
This code ensures that the function is only declared if it doesn’t already exist.
Cache and Reload: After making the necessary changes, clear your browser cache and any caching plugins you may be using. Then, refresh the page to see if the error persists.
Remember that modifying your theme’s functions.php
file incorrectly can lead to site errors. It’s a good practice to make a backup of the file before making any changes and to test changes on a staging or local environment first.